How to setup i18n with Vue & Nuxt3
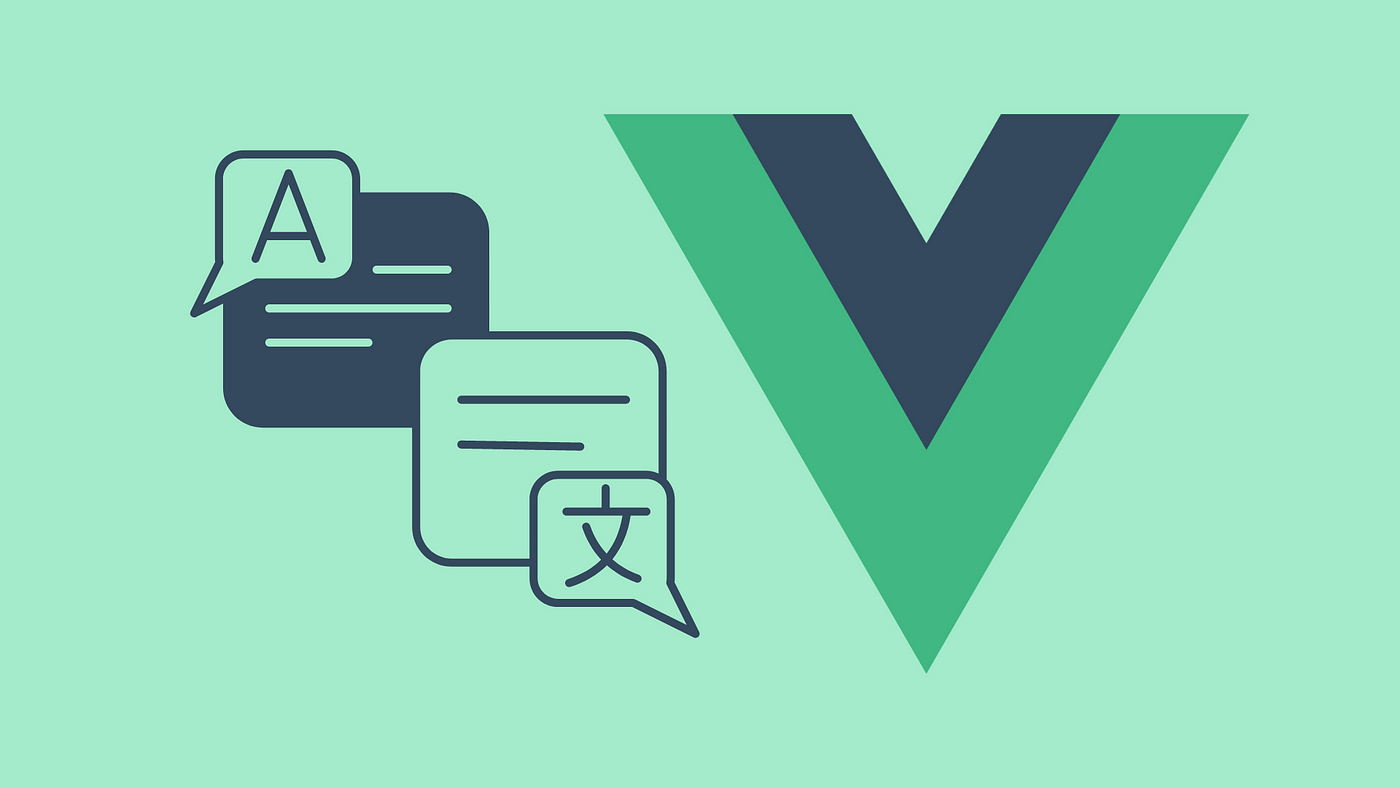
17 Apr 2023
Index
Why do you need i18n?
With i18n you can translate your entire web application in multiple languages and have access to few cool features as:
- Integration with Vue I18n
- Automatic routes generation and custom paths
- Search Engine Optimization
- Lazy-loading of translation messages
- Redirection based on auto-detected language
- Different domain names for different languages
Install and setup i18n
I'm going to use npm
but you could install it with pnpm
or yarn
.
To get started let's install the package:
npm install @nuxtjs/i18n@next --save-dev
Now add @nuxtjs/i18n
to the modules
section in your nuxt.config.js/ts
:
[nuxt.config.js/ts]
export default defineNuxtConfig({
modules: [
'@nuxtjs/i18n',
],
i18n: {
/* module options */
locales: ['en', 'it'], // list of locales in your site
defaultLocale: 'en', // default locale of your site
}
})
I suggest you to use a json
or yaml
file for every language you need. To do this you have to set some options in your i18n options
inside nuxt.config
:
- add
langDir: 'locales/'
- add the list of your languages (
locales: [Array]
):
[nuxt.config.js/ts]
locales: [
{ code: 'it', iso: 'it', file: 'it.json' },
{ code: 'en', iso: 'en-US', file: 'en.json' },
],
* You can find the list of ISO codes here
- create the folder
locales
, as I write after thelangDir
option, in the main path of your project. - create the language file
.json
or.yaml
. Assume that you want to use italian and english as locales, you have to create 2 files nameden.json
andit.json
like this:
[en.json]
{
// "i18n key": "word"
"title": "Welcome",
"desc": "What a wonderful day!"
}
[it.json]
{
"title": "Buongiorno",
"desc": "Che bella giornata oggi!"
}
If you don't have much text you can get rid of using json/yaml file and start using a i18n.config.ts
file.
- create the
i18n.config.ts
file in the main path of your project. - link this file in your
nuxt.config
file by simply adding this linevueI18n: './i18n.config.ts'
:
[nuxt.config.js/ts]
i18n: {
// other options
vueI18n: './i18n.config.ts' // if you are using custom path, default
}
- edit the i18n config file:
[i18n.config.ts]
export default defineI18nConfig(nuxt => ({
legacy: false,
locale: 'en',
messages: {
en: {
"title": "Welcome",
"desc": "What a wonderful day!"
},
it: {
"title": "Buongiorno",
"desc": "Che bella giornata oggi!"
}
}
}))
Start using i18n in your project
Now you can access the i18n keys wherever you want inside your project. Let's try to use the keys I created in the example in a Vue component.
The syntax is $t('YOURKEY')
.
<template>
<div>
<h1>{{ $t('title') }}</h1>
<button>{{ $t('desc') }}</button>
</div>
</template>
<script setup>
//
</script>
In the DOM this result as:
Welcome
What a wonderful day!